Understand how to work with databases in Python from "summary" of Python For Dummies by Stef Maruch,Aahz Maruch
To work with databases in Python, you need to use a database interface module. Several such modules are available, including the Python standard module called DB-API. You can use DB-API to communicate with relational databases that support SQL (Structured Query Language). To use DB-API, you first import it, as you would with any other module in Python. Next, you establish a connection to the database by calling the `connect()` method of the module, passing it the necessary connection parameters, such as the database name, user name, password, and host. Once the connection is established, you create a cursor object by calling the `cursor()` method on the connection object. The cursor object is used to execute SQL statements and retrieve results from the database. To execute SQL statements, you call the `execute()` method on the cursor object, passing it the SQL statement as a string. You can also pass parameters to the SQL statement using placeholders and provide the parameter values as a separate argument to the `execute()` method. After executing a SELECT statement, you can retrieve the results using methods like `fetchone()`, `fetchall()`, or `fetchmany()` on the cursor object. To insert, update, or delete data in the database, you execute SQL statements using the `execute()` method on the cursor object. Remember to commit the transaction by calling the `commit()` method on the connection object after making changes to the database. Lastly, you should always close the cursor and the connection to release database resources by calling the `close()` method on these objects. This ensures that you don't leave any connections open unnecessarily, which could lead to performance issues or resource leaks. By following these steps, you can effectively work with databases in Python using the DB-API module.Similar Posts
Handle missing data effectively
Handling missing data effectively is a crucial aspect of data analysis. When dealing with real-world data, it is common to enco...
SQL is a language for managing relational databases
SQL is indeed a language, but it's not a general-purpose language like C++ or Java. Rather, it's a special-purpose language, sp...
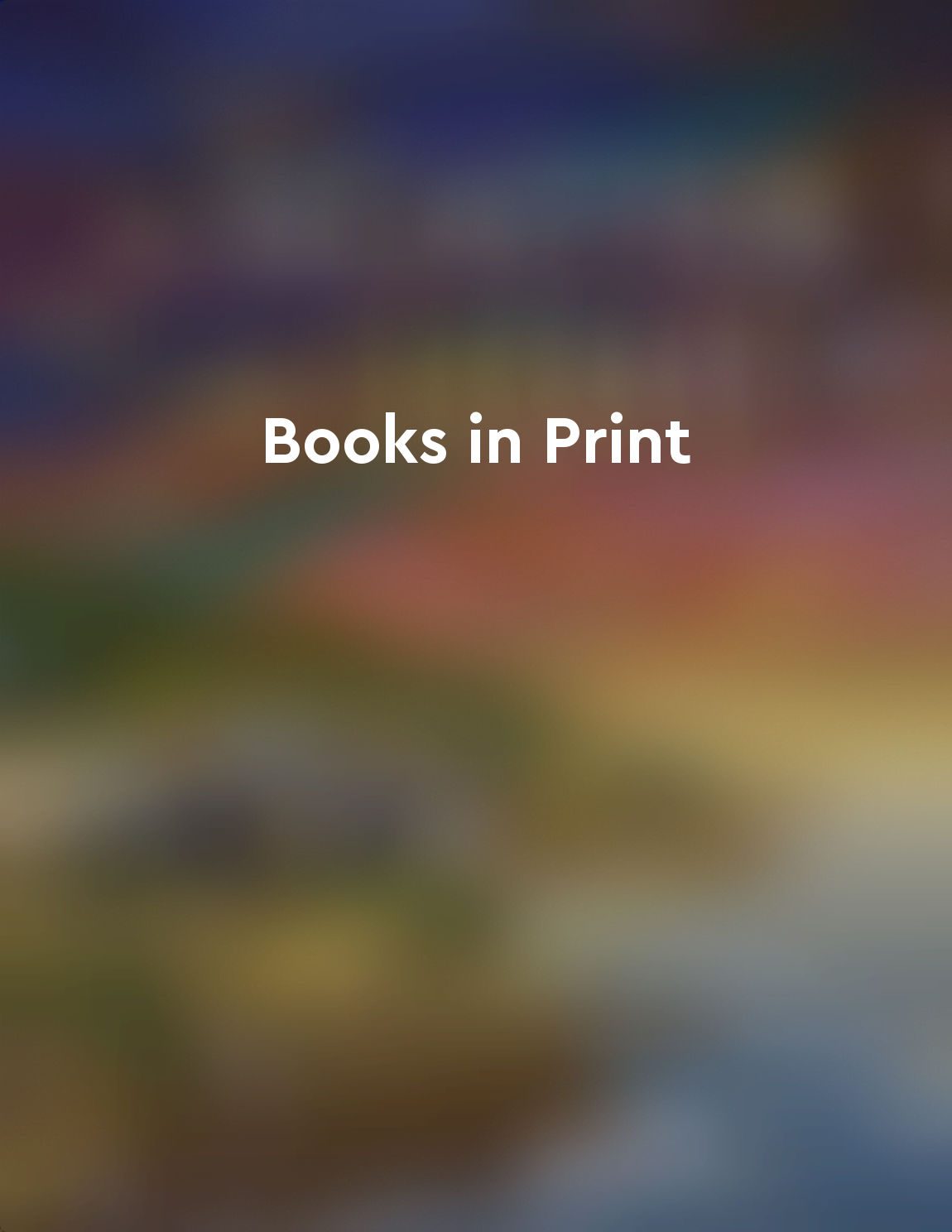
Searchable database
A searchable database is a powerful tool that allows users to quickly and efficiently find relevant information. This type of d...
Inheritance allows classes to inherit attributes and methods from other classes
Inheritance is a fundamental concept in object-oriented programming that allows classes to inherit attributes and methods from ...
Data scientists use Python and R for analysis
Data scientists rely heavily on programming languages like Python and R to carry out their data analysis tasks. These languages...
Understand the importance of testing and debugging in Python
Testing and debugging are crucial aspects of programming in Python. When you write code, it's important to test it thoroughly t...
Stored procedures encapsulate SQL logic
Stored procedures are an essential feature of SQL implementations. The basic idea is to store SQL code on the server, instead o...
Sequences can be sliced and accessed with indices
In Python, sequences such as lists and tuples can be sliced and accessed using indices. Slicing allows you to extract a portion...
Learn how to write and execute Python code
To become proficient in Python, you must first understand the fundamentals of writing and executing code. Python is a high-leve...