Explore functions and recursion from "summary" of C/C++ Programmer's Reference by Herbert Schildt
The concept of functions and recursion is essential in programming. Functions allow you to break down a program into smaller, manageable pieces that can be reused multiple times. By encapsulating code into functions, you can improve the readability and maintainability of your code. Recursion is a powerful technique where a function calls itself to solve a problem. This can be particularly useful in situations where a problem can be broken down into smaller, similar subproblems. Functions in C/C++ are defined using the syntax: return-type function-name(parameters). The return-type specifies the type of value that the function will return. The function-name is the name of the function, which must be unique within the scope it is defined. Parameters are variables that are passed to the function, which can be used within the function body. When defining a function, you need to provide a function prototype before the function is called. This informs the compiler about the function's existence, allowing you to call the function before its definition in the code. Function prototypes are typically placed at the beginning of a C/C++ file or in header files. Recursion is a technique where a function calls itself within its body. This can be useful in solving problems that can be broken down into smaller, similar subproblems. To prevent infinite recursion, you need to include a base case in your recursive function. The base case is a condition that stops the recursion by returning a value without making a recursive call. When using recursion, you need to be mindful of the stack space used by recursive function calls. Each recursive call adds a new stack frame to the call stack, which can lead to a stack overflow if too many recursive calls are made. To prevent this, you can optimize the recursive function or convert it into an iterative solution.- Functions and recursion are fundamental concepts in programming that allow you to break down complex problems into smaller, manageable pieces. By encapsulating code into functions and using recursion when necessary, you can write more readable, maintainable, and efficient code.
Similar Posts
Become familiar with structures and unions
To work effectively in C or C++, you must become proficient with structures and unions. These two features enable you to create...
Inheritance allows classes to inherit attributes and methods from other classes
Inheritance is a fundamental concept in object-oriented programming that allows classes to inherit attributes and methods from ...
Embracing challenges leads to growth
Facing challenges head-on is a crucial aspect of personal growth. When we embrace challenges, we open ourselves up to new oppor...
Python is a popular programming language
Python has gained immense popularity in recent years for a variety of reasons. One of the key factors contributing to its wides...
Maintain a positive attitude towards problemsolving and learning
Having a positive attitude towards problem-solving and learning is crucial for success in mathematical olympiads. When faced wi...
Tips and tricks are shared for efficient problemsolving
Throughout the book, you will find numerous methods and strategies that can help you tackle various arithmetic problems efficie...
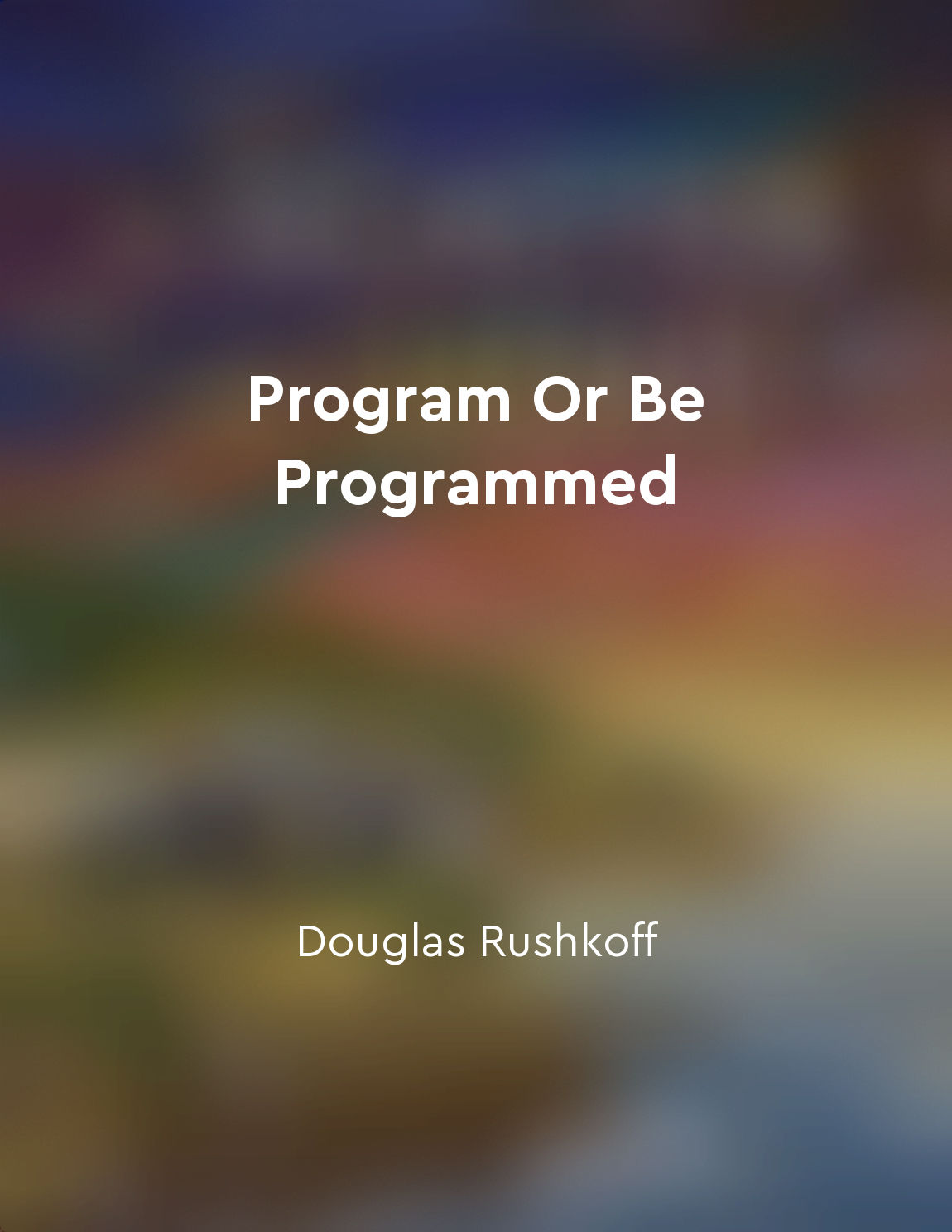
Programming empowers individuals to create and problemsolve
Programming is not just a technical skill; it is a mode of thinking that can empower individuals to create and problem-solve in...
Pythonic code is clear and expressive
Pythonic code is clear and expressive. This clarity is achieved through simplicity in design and implementation. By following P...
Covers all chapters from Class 10 Science syllabus
The content of this book is designed to encompass all the essential topics and concepts from the Class 10 Science syllabus. Eac...
Exploring ratio and proportion
Exploring ratio and proportion involves understanding the relationship between two or more quantities in terms of how they comp...